Flow Control is the sequence of your computer to run your code. Let’s say there is a 5 level platform game in Scratch, we have to go through the level 1 before we go to the next level right? The computer will run the first block of your code then run the next one until the end. Unless you fail the platform game before it gets to the end. In this lesson, we are going to learn the statement that controls the flow of our code.
If Statements
💭 Refresher on if
statements: We use if statement to check the condition of the code is true or not, if it’s true, we execute the code. If it’s not, we ignore it.
Comparison Operators
- Equality operator (
==
) - Less than / less than or equal (
<
/<=
) - More than / more than or equal (
>
/>=
) - Inequality operator (
!=
)
If-else Statements
Sometimes you want to continue and do something else if whatever comes out isn’t true.
To do that, you can add an else at the end.
if (condition) {
// branch 1
} else {
// branch 2
}
Here is an example from Scratch!
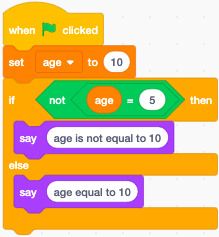
If-else Chain Statements
Here we use the If-else Chain statement, we have different classes below, when the computer runs the code, it will find which one matches the value “Math”. You must also notice == in the code, this is Equality operators, this means to match the content and also have the same data type as the variable value!
let classes = "Math";
if (classes == "Math") {
alert("Math Class");
} else if (classes == "Sport") {
alert("Sport Class");
} else if (classes == "Music") {
alert("Music Class");
} else if (classes == "Science") {
alert("Science Class");
} else {
alert("Unknow Classes");
}
Logical Operators
Logical operators are used to verifying the logic between variables or values.
Operator | Description | Examples |
&& | and | (x > 5 && y < 6) is true |
|| | or | (x == 5 || x > 6) is true |
! | not | !(x == y) is true |
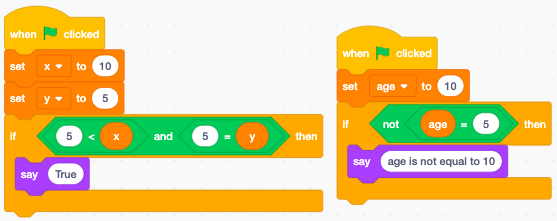
Switch Statements
In Switch Statement, the value of the variable can be any data type, we can have different numbers of the case following with different values. The break here it’s to tell the program that we meet the condition and can jump out of the switch statement.have to exit) When every case is not equal to the variable value, then we run the default value. (Default is not necessarily existed)
let fruite = "Apple";
switch (fruite) {
case "Banana":
alert("Hello");
break;
case "Apple":
alert("Welcome");
break;
default:
alert("Don't have the fruit!");
break;
}